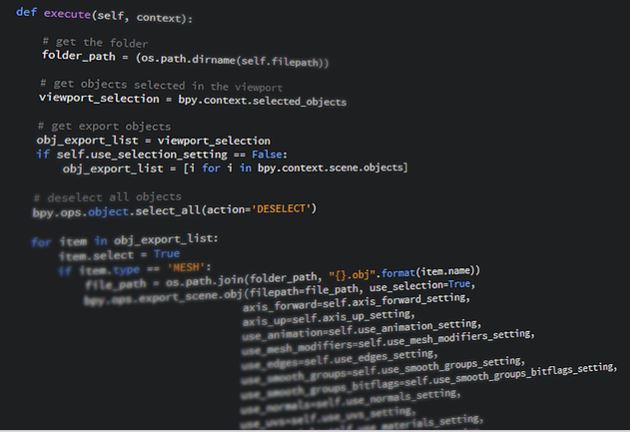
We have received few request related to Python Program, So here we are with list of most asked Python Program.
- Write a Python program to add , Subtract, Multiply, and divide 2 numbers.
- Write a Python program to find the biggest of 3 numbers ( Use If Condition )
- Write a Python program to find given number is odd or Even
- Write a Python program to find the number is Prime or not.
- Write a Python program to receive 5 command line arguments and print each argument separately.
Example :
>> python test.py arg1 arg2 arg3 arg4 arg5
a) From the above statement your program should receive arguments and print them each of them.
b) Find the biggest of three numbers, where three numbers are passed as command line arguments. - Write a Python program to read string and print each character separately.
a) Slice the string using slice operator [:] slice the portion the strings to create a sub strings.
b) Repeat the string 100 times using repeat operator *
c) Read strig 2 and concatenate with other string using + operator. - Create a list with at least 10 elements in it
print all elements
perform slicing
perform repetition with * operator
Perform concatenation wiht other list. - Repeat program 7 with Tuple( Take example from Tutorial )
- Write program to Add , subtract, multiply, divide 2 complex numbers.
- Using assignment operators, perform following operations
Addition, Substation, Multiplication, Division, Modulus, Exponent and Floor division operations - Read 2 numbers to variable a and b and perform all bitwise operations on that numbers.
- Read 10 numbers from user and find the average of all.
a) Use comparison operator to check how many numbers are less than average and print them
b) Check how many numbers are more than average.
c) How many are equal to average. - Write a program to find the biggest of 4 numbers.
a) Read 4 numbers from user using Input statement.
b) extend the above program to find the biggest of 5 numbers.
( PS : Use IF and IF & Else , If and ELIf, and Nested IF ) - Write a program to find the biggest of 4 numbers.
a) Read 4 numbers from user using Input statement.
b) extend the above program to find the biggest of 5 numbers.
( PS : Use IF and IF & Else , If and ELIf, and Nested IF ) - Create a list of 5 names and check given name exist in the List.
a) Use membership operator ( IN ) to check the presence of an element.
b) Perform above task without using membership operator.
c) Print the elements of the list in reverse direction. - Write program to perform following:
i) Check whether given number is prime or not.
ii) Generate all the prime numbers between 1 to N where N is given number. - Write program to find the biggest and Smallest of N numbers.
PS: Use the functions to find biggest and smallest numbers. - Using loop structures print numbers from 1 to 100. and using the same loop print numbers from 100 to 1.( reverse printing)
a) By using For loop
b) By using while loop
c) Let mystring =”Hello world”
print each character of mystring in to separate line using appropriate loop structure. - Using loop structures print even numbers between 1 to 100.
a) By using For loop , use continue/ break/ pass statement to skip odd numbers.
i) break the loop if the value is 50
ii) Use continue for the values 10,20,30,40,50
b) By using while loop, use continue/ break/ pass statement to skip odd numbers.
i) break the loop if the value is 90
ii) Use continue for the values 60,70,80,90 - Write a program to generate Fibonacci series of numbers.
Starting numbers are 0 and 1, new number in the series is generated by adding previous two numbers in the series.
Example : 0, 1, 1, 2, 3, 5, 8,13,21,…..
a) Number of elements printed in the series should be N numbers, Where N is any +ve integer.
b) Generate the series until the element in the series is less than Max number.
Solutions
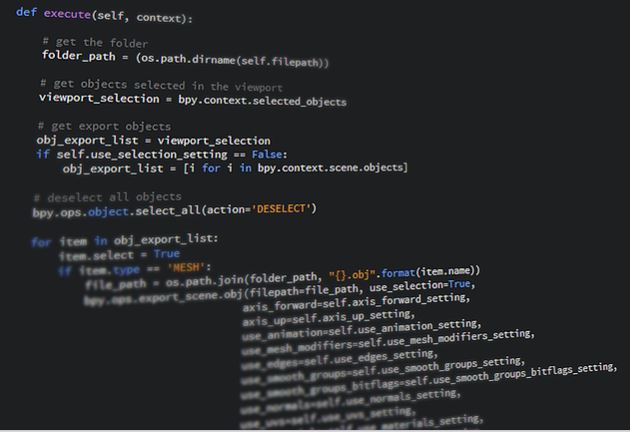
1. Write a program to add , Subtract, Multiply, and divide 2 numbers
a=20
b=10
c=a+b
d=a-b
f=a*b
e=a/b
print”The addition of the two numbers %d and %d is %d”%(a,b,c)
print”Thesubstraction of the two numbers %d and %d is %d”%(a,b,d)
print”The multiplication of the two numbers %d and %d is %d”%(a,b,f)
print”Thedivison of the two numbers %d and %d is %d”%(a,b,e)
2. Write a program to find the biggest of 3 numbers ( Use If Condition )
a=input(“Enter a value for a: “)
b=input(“Enter a value for b: “)
c=input(“Enter a value for c: “)
if a>b and a>c:
print “a is the greatest number”
elif b>a and b>c:
print “b is the greatest number”
else:
print”c is the greatest number”
3. Write a program to find given number is odd or Even
a=input(“Enter a value for a: “)
if (a%2==0):
print”a is a even number”
else:
print”a is a odd number”
4. Write a program to find the number is Prime or not.
a=input(“enter a number: “)
k=0
fori in range(2,a):
k=k+1
if(k<=0):
print”prime“
print a
else:
print”Not prime”
5. Write a program to receive 5 command line arguments and print each argument separately.
Example :
# >> python test.py arg1 arg2 arg3 arg4 arg5
- a) From the above statement your program should receive arguments and print them each of them.
from sys import argv
print dir()
print”The values entered in the Command Line Arguments is”,argv
i=0
for i in range(o,len(argv)):
print”argv[%d]: %s”%(i,argv[i])
i=i+1
print”End of the program”
- b) Find the biggest of three numbers, where three numbers are passed as command line arguments
from sys import argv
print”The value entered in the command line argument is”,argv
rom sys import argv
if eval(argv[1])> eval(argv[2]) and eval(argv[1])>eval( argv[3]):
print”argv[0] is greatest:”,eval(argv[0])
elifeval(argv[2])>eval(argv[3]) and eval (argv[2])> eval(argv[1]):
print”argv[2] is greatest:”,eval(argv[2])
else:
print”argv[3] is greatest:”,eval(argv[3])
6. a) Slice the string using slice operator [:] slice the portion the strings to create a sub strings.
sub_str=str[0:4]
print sub_str
for i in range(0,len(sub_str)):
print sub_str[i]
6.BRepeat the string 100 times using repeat operator *
print str*100
6.CRead strig 2 and concatenate with other string using + operator.
str2=raw_input(“Enter a another string: “)
str3=str +str2
print str3
7. Create a list with at least 10 elements in it
print all elements
perform slicing
perform repetition with * operator
Perform concatenation wiht other list.
list1=[1,2,3,4,5,”Bittu”,6,7,”Dey”,’d’]
fori in range(0,len(list1)):
print list1[i]
SList=list1[0:5]
printSList
8. Repeat program 7 with Tuple( Take example from Tutorial )
Tuple1=(1,2,3,4,5,”Bittu”,6,7,”Dey”,’d’)
fori in range(0,len(Tuple1)):
print Tuple1[i]
STuple=Tuple1[0:5]
printSTuple
printSTuple*10
Tuple2=(“Ram”,”shyam“,”Ghanshayam“)
CTuple=Tuple1 + Tuple2
printCTuple
printSList*10
List2=[“Ram”,”shyam“,”Ghanshayam“]
CList=list1 + List2
printCList
9. Write program to Add , subtract, multiply, divide 2 complex numbers.
importcmath
x=10
y=20
a=complex(x,y)
c=5
d=10
b=complex(c,d)
print”The additions of the two complex number”,a+b
print”Thesubstraction of the two complex number”,a-b
print”The multiplication of the two complex number”, a*b
print”Thedivison of the two complex number”, a/b
printa.real
printa.imag
10. Using assignment operators, perform following operations
Addition, Substation, Multiplication, Division, Modulus, Exponent and Floor division operations
a=input(“Enter a value for a:-“)
b=input(“Enter a value for b:-“)
print”The additions of the two number”,a+b
print”Thesubstraction of the two number”,a-b
print”The multiplication of the two number”, a*b
print”Thedivison of the two number”, a/b
print”The modulus of the two numbers is”,a%b
print “The exponent of the two number is “,pow(a,b)
11. Read 2 numbers to variable a and b and perform all bitwise operations on that numbers.
a=input(“Enter a value for a”)
b=input(“Enter a value for b”)
printa&b
printa|b
printa^b
print ~a
print a>>2
print a<<2
12. Write a program to find the biggest of 4 numbers.
a) Read 4 numbers from user using Input statement.
b)extend the above program to find the biggest of 5 numbers.
( PS : Use IF and IF & Else , If and ELIf, and Nested IF )
13.a
a=input(“Enter a number: “)
b=input(“Enter a number: “)
c=input(“Enter a number: “)
d=input(“Enter a number: “)
if(a>b and a>c and a>d):
print”A is the greatest number”
elif(b>c and b>d and b>a):
print”B is the greatest”
elif(c>a and c>b and c>d):
print”C is the greatest”
else:
print”D is the greatest”
13.b
a=input(“Enter a number: “)
b=input(“Enter a number: “)
c=input(“Enter a number: “)
d=input(“Enter a number: “)
e=input(“Enter a number: “)
if(a>b and a>c and a>d and a>e):
print”A is the greatest number”
elif(b>c and b>d and b>a and b>e):
print”B is the greatest”
elif(c>a and c>b and c>d and c>e):
print”C is the greatest”
elif(d>a and d>b and d>c and d>e):
print”D is the greatest”
else:
print”E is the greatest”
14.Write a program to create two list A and B such that List A contains Employee Id, List B contains Employee name
( minimum 10 entries in each list ) and perform following operations
a) Print all names on to screen
b) index from the user and print Read the the corresponding name from both list.
c) Print the names from 4th position to 9th position
d) Print all names from 3rd position till end of the list
e) Repeat list elements by specified number of times ( N- times, where N is entered by user)
f) Concatenate two lists and print the output.
g) Print element of list A and B side by side.( e. List-A First element , List-B First element )
list1=[12,24,3,4,5,6,7,8,9,10]
list2=[“A”,”B”,”C”,”D”,”E”,”F”,”G”,”H”,”I”,”J”]
a.
print list2
b.
Index=input(“Enter the index”)
if Index>9:
print”Please enter a index between less than 9 to 0″
elif Index<0:
print”Please enter a index which is greater than or equal to 0″
else:
for i in (0,len(B)):
i=Index
print B[i]
c.
print list2[4:10]
d.
print list2[3:]
e.
N=input(“Enter the number of repetation“)
print list2*N
f.
List3=list1+list2
print List3
g.Print element of list A and B side by side.( i.e. List-A First element , List-B First element )
print zip(Lis1,List2)
15. Create a list of 5 names and check given name exist in the List.
a) Use membership operator( IN ) to check the presence of an element.
b) Perform above task without using membership operator.
c) Print the elements of the list in reverse direction.”””
list1=[“Ram”,”Shyam“,”Bittu“,”Rahul”,”Shiv“]
#a.
if “Ram” in list1:
print “Ram exists in list1”
else:
print”ram do not exists in list1″
#b.
if list1.count(“Ram”)>0:
print”Ram exists in list1″
else:
print”Ram do not exists in list1″
#c.
list1.reverse()
print list1
16. Write program to perform following:
- i) Check whether given number is prime or not.
- ii) Generate all the prime numbers between 1 to N where N is given number.
#16.1
a=int(input(“Enter number: “))
k=0
fori in range(2,a-1):
if(a%i==0):
k=k+1
if(k<=0):
print(“Number is prime”)
print a
else:
print(“Number isn’t prime”)
17. Write program to find the biggest and Smallest of N numbers.
PS: Use the functions to find biggest and smallest numbers.
lst = []
num = int(input(‘How many numbers: ‘))
for n in range(num):
numbers = int(input(‘Enter number ‘))
lst.append(numbers)
print(“Maximum element in the list is :”, max(lst), “\nMinimum element in the list is :”, min(lst))
18. Using loop structures print numbers from 1 to 100. and using the same loop print numbers from 100 to 1.( reverse printing)
- a) By usingFor loop
- b) By using while loop
- c) Let mystring =”Hello world”
print each character of mystring in to separate line using appropriate loop structure.
#18.a
for i in range(1,100,+1):
print i
for b in range(100,0,-1):
print b
#18.b
i=1
while(i<101):
print i
i=i+1
i=100
while(i>0):
print i
i=i-1
#18.c
mystring=”Hello World”
fori in range(0,len(mystring)):
printmystring[i]
19.Using loop structures print even numbers between 1 to 100.
- a) By using Forloop , use continue/ break/ pass statement to skip odd numbers.
- i) break the loop if the value is 50
- ii) Use continue for the values 10,20,30,40,50
- b) By using while loop, use continue/ break/ pass statement to skip odd numbers.
- i) break the loop if the value is 90
- ii) Use continue for the values 60,70,80,90
#19.a)i)
for i in range(1,100):
if i%2==0:
print i
if i==50:
print”The loop terminates and control has been given to the next line”
break
else:
pass
#19.a)ii)
for i in range(1,100):
if i%2==0:
if i==10:
print”The Control is given back to the top of the loop”
continue
if i==20:
print”The Control is given back to the top of the loop”
continue
if i==30:
print”The Control is given back to the top of the loop”
continue
if i==40:
print”The Control is given back to the top of the loop”
continue
if i==50:
print”The Control is given back to the top of the loop”
continue
print i
else:
pass
#19.b.i
i=1
while(i<100):
i=i+1
if i%2==0:
print i
if i==90:
break
#19.b.ii
i=1
while(i<100):
i=i+1
if i%2==0:
ifi==60:
continue
ifi==70:
continue
ifi==80:
continue
ifi==90:
continue
printi
20.Write a program to generate Fibonacci series of numbers.
Starting numbers are 0 and 1, new number in the series is generated by adding previous two numbers in the series.
Example : 0, 1, 1, 2, 3, 5, 8,13,21,…..
- a)umber of elements printed in the series should be N numbers, Where N is any +ve N
- b) Generate the series until the element in the series is less than Max number.
nterms=input(“Enter the terms: “)
n1=0
n2=1
count=0
ifnterms<=0:
print(“Please enter a positive number”)
elifnterms==1:
print”Fibonacci series upto:”,nterms,”:”
print n1
else:
print”Fibonacci series upto:”,nterms,”:”
while(count<=nterms):
print n1
nth=n1+n2
n1=n2
n2=nth
count=count +1
n=input(“Enter a number:”)
fact=1
for a in range(n,n+1):
fact=fact*a
a=a-1